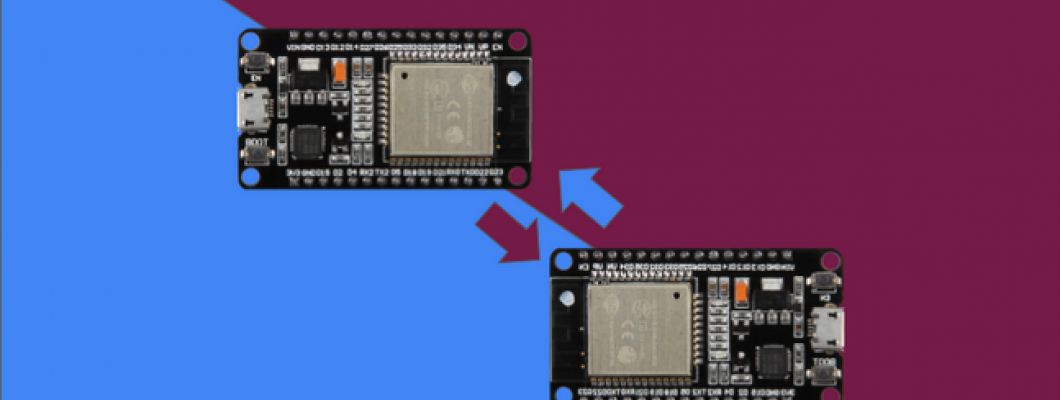
Welcome to a new tutorial on Google Cloud Platform and ESP32. In this blog we are establishing a device to device communication via GCP. The system consists of two ESP32 boards of which one has a relay board connected to it which is toggled using the in-built button of the other board.
PINOUT
We will be using the built-in push button of the ESP32 module for this tutorial with a Relay module getting toggled along with the button.
HARDWARE CONNECTION
A 5V relay is used here and hence, assuming that a sufficient power supply is given to the ESP32 module, the relay is directly connected to the Vin of the module.
The second ESP32 board has no external peripherals connected as only the built-in button is used in this tutorial.
GOOGLE IOT CORE ESP32 SETUP
Follow the previous blog for set up instructions. The link is given below:
https://www.elementzonline.com/blog/Connecting-ESP32-to-Google-Cloud-IoT
If you have already completed the setup, you can continue to the next step.
WRITING THE CLOUD FUNCTION
First open the GCP console
Now, navigate to the Cloud Functions page. (You can use the search tool)
Click the Create Function button.
Now fill in a preferred function name (Eg: relaycloudiot) and your region (Eg: europe-west1). Select the trigger type as Cloud Pub/Sub and then select the topic to which your devices were added during the setup.
Click save and proceed to the next step of uploading the code.
A new window will appear. Select the Runtime as Node.js 10 and Entry Point as recvMsg
Select the index.js file and paste the below code into the editor.
Now select the package.json file and paste the code below into the editor.
Finally use the deploy button to deploy your function
Wait till the green tick mark appears.
TRANSMITTER ESP32 SETUP
Use the Mongoose OS Tool to upload the code below to the ESP32 module.
To know how visit the previous blog:
https://www.elementzonline.com/blog/Connecting-ESP32-to-Google-Cloud-IoT
On successful upload, you should be able to start seeing button at the Serial Console of the MOS Tool.
RECEIVER ESP32 SETUP
Use the Mongoose OS Tool to upload the code below to the ESP32 module with the Relay board connected. [P.S. Select the correct COM Port for the ESP32 module, if both the modules are connected to the same PC.]
If everything was set up correctly, you should be able to toggle the relay connected at the receiver ESP32 module with the in-built button of the transmitter ESP32 module.
You can use the serial console of mongoose to verify the same.