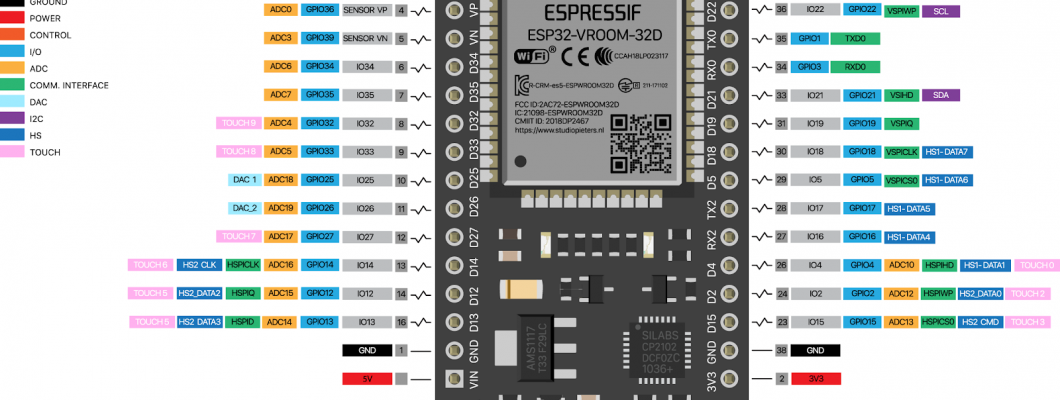
In the previous blog we discussed connecting ESP32 to the Google Cloud Platform (GCP). Now, In this tutorial we will learn how to establish a communication between two ESP32 boards connected to GCP. The tutorial consists of a ESP32 board connected with a DHT11 sensor, publishing data which is received by the second ESP32 board. A Cloud Function written in GCP acts as a bridge to facilitate the communication.
PINOUT
We will be using the built-in LED and push button of the ESP32 module for this tutorial with a DHT11 sensor monitoring the temperature and humidity.
HARDWARE CONNECTION
The second ESP32 board has no external peripherals connected as only the built-in LED is used in this tutorial.
GOOGLE IOT CORE ESP32 SETUP
Follow the previous blog for set up instructions. The link is given below:
https://www.elementzonline.com/blog/Connecting-ESP32-to-Google-Cloud-IoT
If you have already completed the setup, you can continue to the next step.
WRITING THE CLOUD FUNCTION
First open the GCP console
Now, navigate to the Cloud Functions page. (You can use the search tool)
Click the Create Function button.
Now fill in a preferred function name (Eg: relaycloudiot) and your region (Eg: europe-west1). Select the trigger type as Cloud Pub/Sub and then select the topic to which your devices were added during the setup.
Click save and proceed to the next step of uploading the code.
A new window will appear. Select the Runtime as Node.js 10 and Entry Point as recvMsg
Select the index.js file and paste the below code into the editor.
Now select the package.json file and paste the code below into the editor.
Finally use the deploy button to deploy your function
Wait till the green tick mark appears.
TRANSMITTER ESP32 SETUP
Use the Mongoose OS Tool to upload the code below to the ESP32 module with the DHT11 Sensor Connected.
To know how visit the previous blog:
https://www.elementzonline.com/blog/Connecting-ESP32-to-Google-Cloud-IoT
On successful upload, you should be able to start seeing the temperature and humidity values at the Serial Console of the MOS Tool.
RECEIVER ESP32 SETUP
Use the Mongoose OS Tool to upload the code below to the second ESP32 module. [P.S. Select the correct COM Port for the ESP32 module, if both the modules are connected to the same PC.]
If everything was set up correctly, you should be able to receive the temperature and humidity values from the first ESP32 module and should be printed on the Serial Console.
Also, if the temperature increases more than 30 degree celsius, the built-in LED of the module will turn on.