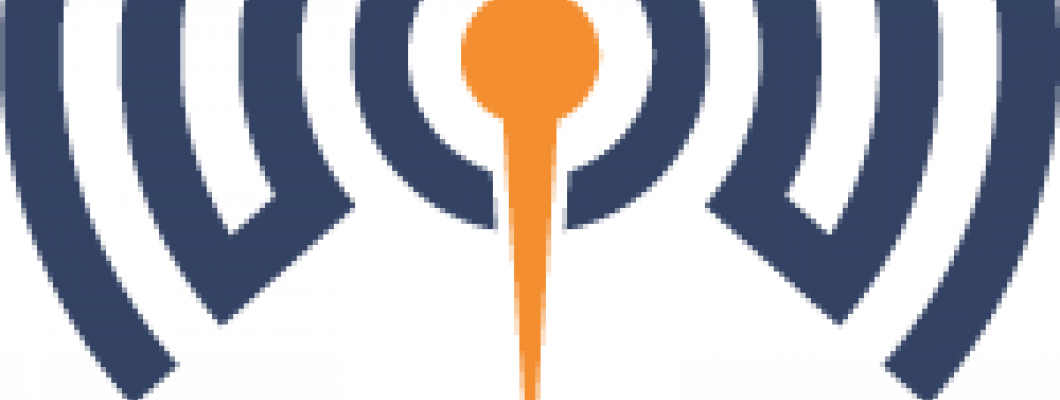
Today we are going to discuss implementing our own MQTT broker in RaspberryPi. We will be using Mosquitto as the MQTT broker. Mosquitto is an open source message broker that implements the MQTT (MQ Telemetry Transport) protocol v3.1.
Use cases:
- Create our own home automation hub by interconnecting various MQTT enabled devices.
- Create a central hub which can be used as a bridge to connect to remote locations.
Using raspberry pi as a HUB has the following advantages
- We can provide SSL certificates to provide the bridge authentication for secure connectivity to the remote servers.
- Using a central hub provides easy scalability and security
- Many automation processes can be easily achieved even if there is no network access
As the first step, we will be running an MQTT broker in Raspberry Pi which can be used to connect to the local wifi devices. For simplicity, we will not be using advanced authentication techniques provided by the Mosquitto broker. Instead will stick on with the default port 1883 and without username and password for connection by the client
sudo apt-get install mosquitto
The configuration will be saved in /etc/mosquitto/mosquitto.conf
You can make changes if required. But the server should be restarted making changes.
sudo service mosquitto restart
Install MQTT client library using the following command.
sudo pip install paho-mqtt
Check whether MQTT server is running using the following command
sudo netstat -l -t
Following programs can be used for testing. It's better to assign static ip to your pi, as the ip will be required to configure the devices to connect to your raspberry pi's MQTT server. test_publish.py
'''
Developed By: Dhanish Vijayan
Company: Elementz Engineers Guild Pvt Ltd
'''
import paho.mqtt.client as mqtt
mqttc = mqtt.Client("python_pub")
mqttc.connect("192.168.1.202", 1883) # use the ip of your rpi here
mqttc.publish("Elementz/welcome", "Welcome to Open Source")
mqttc.loop(2) #timeout = 2s
test_subscibe.py
# -*- coding: utf-8 -*-
'''
Developed By: Dhanish Vijayan
Company: Elementz Engineers Guild Pvt Ltd
'''
import paho.mqtt.client as mqtt
# The callback for when the client receives a CONNACK response from the server.
def on_connect(self, client, userdata, rc):
print("Connected with result code "+str(rc))
# Subscribing in on_connect() means that if we lose the connection and
# reconnect then subscriptions will be renewed.
self.subscribe("Elementz/welcome")
# The callback for when a PUBLISH message is received from the server.
def on_message(client, userdata, msg):
print "Topic: ", msg.topic+'\nMessage: '+str(msg.payload)
client = mqtt.Client()
client.on_connect = on_connect
client.on_message = on_message
client.connect("192.168.1.202", 1334, 60)
# Blocking call that processes network traffic, dispatches callbacks and
# handles reconnecting.
# Other loop*() functions are available that give a threaded interface and a
# manual interface.
client.loop_forever()